在glib中,GHashTable结构的官方文档参看:https://docs.gtk.org/glib/struct.HashTable.html
代码ghash.c
// gcc -o test ghash.c -I/usr/include/glib-2.0 -I/usr/lib/x86_64-linux-gnu/glib-2.0/include -I/usr/include/sysprof-6 -pthread -lglib-2.0
#include <glib.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 键和值的销毁函数
static void string_destroy(gpointer data) {
g_free(data);
}
static void print_hash_node(gpointer key, gpointer value, gpointer user_data) {
printf("key[%s], value[%s], user_data[%s]\n", key, value, user_data) ;
}
int main() {
// 创建哈希表,键和值都是字符串,并指定销毁函数
GHashTable *hash_table = g_hash_table_new_full(g_str_hash, g_str_equal, string_destroy, string_destroy);
// 插入键值对
g_hash_table_insert(hash_table, g_strdup("key1"), g_strdup("value1"));
g_hash_table_insert(hash_table, g_strdup("key2"), g_strdup("value2"));
g_hash_table_insert(hash_table, g_strdup("key3"), g_strdup("value3"));
// 查找键对应的值
gchar *value = g_hash_table_lookup(hash_table, "key2");
if (value) {
g_print("Found key2: %s\n", value);
} else {
g_print("key2 not found\n");
}
// 更新键对应的值
g_hash_table_replace(hash_table, g_strdup("key2"), g_strdup("new_value2"));
value = g_hash_table_lookup(hash_table, "key2");
if (value) {
g_print("Updated key2: %s\n", value);
}
// 删除键值对
g_hash_table_remove(hash_table, "key1");
value = g_hash_table_lookup(hash_table, "key1");
if (!value) {
g_print("key1 removed\n");
}
// 遍历哈希表并打印所有键值对
g_hash_table_foreach(hash_table, (GHFunc)print_hash_node, "HHHHHHH");
g_print("\n");
// 销毁哈希表(这将自动调用键和值的销毁函数)
g_hash_table_destroy(hash_table);
return 0;
}
编译测试
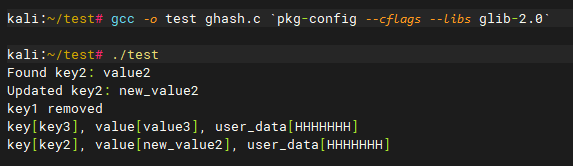
发表回复