minio关于python的sdk,参看:https://min.io/docs/minio/linux/developers/python/API.html
minio的基本操作,参看往期文章:https://www.madbull.site/?p=792
文件名 minio_client.py
代码如下,需要替换 endpoint、accessKeyId、secretAccessKey、usessl 的值。
# -*- coding: utf-8 -*-
# minio_client.py
from minio import Minio
import io
import os
// bucket前缀,根据日期自动生成bucket名字
bucket_name_pre = "test-"
endpoint = "HOST:PORT"
accessKeyId = "accesskey"
secretAccessKey = "secretkey"
usessl=False
// 客户端连接
minio_client=Minio(endpoint, access_key=accessKeyId, secret_key=secretAccessKey, secure=usessl )
// 如果没有bucket则自动生成bucket
def make_bucket_if_not_exists(bucket_name) :
if minio_client.bucket_exists(bucket_name) :
print("found bucket success : "+bucket_name)
return True
minio_client.make_bucket(bucket_name)
print("make bucket : "+bucket_name)
return True
// 保存文件到minio
def save_to_minio( filename: str, date: str, filecontent: bytes ) :
bucket_name = bucket_name_pre + date[:6]
if make_bucket_if_not_exists(bucket_name) :
rslt = minio_client.put_object(bucket_name, filename, data=io.BytesIO(filecontent), length=len(filecontent))
print(rslt.object_name + " save success!")
return True
else :
return False
// 下载文件到指定目录
def download_from_minio( filename: str, date: str, downloadpath: str ) :
bucket_name = bucket_name_pre + date[:6]
fullpath = os.path.join(downloadpath, filename)
rslt = minio_client.fget_object(bucket_name, filename, file_path=fullpath)
print(rslt.object_name + " download success!")
return True
// 测试
if __name__ == "__main__" :
save_to_minio("tt.txt", "20240822191919", b"aaaaaaaabbbbbbbbbbbccccccccdddddddd\n")
download_from_minio("tt.txt", "20240822191919", "/tmp")
测试结果:
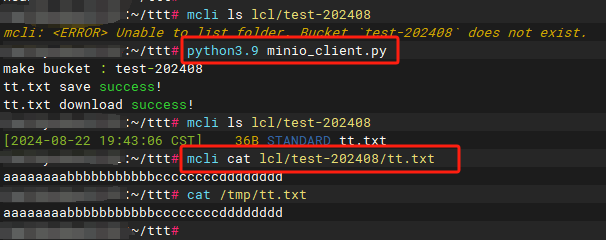
对于加密通讯的minio服务处理方法:
1、忽略证书
// 客户端连接
minio_client=Minio(endpoint, access_key=accessKeyId, secret_key=secretAccessKey, secure=True, cert_check=False )
2、配置信任证书
ssl_context = ssl.create_default_context(cafile="/xxx/xxx/myCA.crt")
http_context = urllib3.PoolManager(ssl_context=ssl_context)
// 客户端连接
minio_client=Minio(endpoint, access_key=accessKeyId, secret_key=secretAccessKey, secure=True, http_client=http_context, cert_check=True )
发表回复