pcre基本使用参看往期文章:https://www.madbull.site/?p=1648 C语言中的正则表达式匹配
说明:
在 PCRE2 库中,JIT 用于优化正则表达式的匹配性能。通过 JIT 编译,正则表达式可以在运行时被转换为机器码,从而显著加快匹配速度。
使用方法:
1、在模式编译后,启动 JIT 编译功能;
2、申请 JIT 栈,分配给匹配上下文;
3、使用匹配上下文对被检测字符串进行匹配。
测试代码 tpjit.c:
// gcc -Wall -o test tpjit.c -lpcre2-8
#ifndef PCRE2_CODE_UNIT_WIDTH
#define PCRE2_CODE_UNIT_WIDTH 8
#endif
#include <stdio.h>
#include <string.h>
#include <pcre2.h>
int main() {
// 1、创建编译上下文
pcre2_compile_context *compile_context = pcre2_compile_context_create(NULL);
// 设置换行符类型为任何 Unicode 换行符
int result = pcre2_set_newline(compile_context, PCRE2_NEWLINE_ANY);
// 2、编译正则表达式
const char *pattern = "a?b(cd|gg)ef";
int errorcode;
PCRE2_SIZE erroroffset;
pcre2_code *re_code = pcre2_compile((PCRE2_SPTR8)pattern, PCRE2_ZERO_TERMINATED, PCRE2_DOTALL|PCRE2_CASELESS, &errorcode, &erroroffset, compile_context);
if (!re_code) {
fprintf(stderr, "Failed to compile pattern\n");
pcre2_compile_context_free(compile_context);
return 1;
}
// 3、启用 JIT 编译
result = pcre2_jit_compile(re_code, PCRE2_JIT_COMPLETE);
// 4、创建 JIT 栈
pcre2_jit_stack *jit_stack = pcre2_jit_stack_create(128 * 1024, 1024 * 1024, NULL);
// 5、创建匹配上下文
pcre2_match_context *match_context = pcre2_match_context_create(NULL);
// 6、给匹配上下文分配 JIT 栈
pcre2_jit_stack_assign(match_context, NULL, jit_stack);
// 创建匹配结果数据
pcre2_match_data *match_data = pcre2_match_data_create_from_pattern(re_code, NULL);
// 7、匹配目标字符串
const char *subject = "uiuiuiuibcDEFhijabCDefklmn";
result = pcre2_match(re_code, (PCRE2_SPTR)subject, strlen(subject), 0, 0, match_data, match_context);
if (result >= 0) {
// 匹配成功,解析检测结果
PCRE2_SIZE *ovector = pcre2_get_ovector_pointer(match_data);
for (int i = 0; i < result; i++) {
printf("match %d: '%.*s'\n", i, (int)(ovector[2 * i + 1] - ovector[2 * i]), subject + ovector[2 * i]);
}
} else {
// 处理错误
fprintf(stderr, "Failed to match pattern\n");
}
subject = "123456abcDEFhijklmn";
result = pcre2_match(re_code, (PCRE2_SPTR)subject, strlen(subject), 0, 0, match_data, match_context);
if (result >= 0) {
// 匹配成功,解析检测结果
PCRE2_SIZE *ovector = pcre2_get_ovector_pointer(match_data);
for (int i = 0; i < result; i++) {
printf("match %d: '%.*s'\n", i, (int)(ovector[2 * i + 1] - ovector[2 * i]), subject + ovector[2 * i]);
}
} else {
// 处理错误
fprintf(stderr, "Failed to match pattern\n");
}
// 清理资源
pcre2_match_data_free(match_data);
pcre2_match_context_free(match_context);
pcre2_jit_stack_free(jit_stack);
pcre2_code_free(re_code);
pcre2_compile_context_free(compile_context);
return 0;
}
编译和测试:
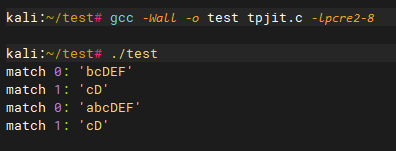
发表回复